Containerize an application using Dockerfile

Docker
Docker is an open platform for developing, shipping, and running applications. Docker enables you to separate your applications from your infrastructure so you can deliver software quickly.
Image:
Image is a blueprint of an application created using Dockerfile, these images are tagged appropriately and stored in repositories using hosted repository services like DockerHub, AWS Elastic Container Registry, etc.
Container:
A container is a single running instance of an application(Docker image).
Dockerfile:
The Dockerfile provides the instructions to build a container image through the command.
It starts from a previously existing Base image (through the FROM clause) followed by any other needed Dockerfile instructions.
Dockerfile Instructions:
Below are the few commonly used dockerfile instructions,
FROM- Sets the base image for the execution of subsequent instructions.
RUN- Execute commands in a new layer on top of the current image and commits the results.
CMD- Command to execute in the base image(Allowed only once).
EXPOSE- Informs the container runtime that the container listens to the specified network ports at runtime.
ENV- Sets an environment variable.
ADD- Copy new files and directories into the filesystem of the container.
COPY- Copy new files or directories into the filesystem of the container.
ENTRYPOINT- This allows you to configure a container that will run as an executable.
WORKDIR- Sets the working directory for any RUN, CMD, ENTRYPOINT, COPY, and ADD commands.
Example
The following dockerfile containerizes a Python Flask application.
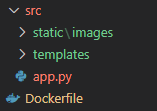
Dockerfile:
FROM python:3.7.0-alpine3.8
WORKDIR /app
COPY requirements.txt /app/requirements.txt
COPY src /app/src
RUN pip install -r requirements.txt
ENV FLASK_APP=src/app.py
ENV FLASK_RUN_PORT=8000
ENV FLASK_RUN_HOST=0.0.0.0
EXPOSE 8000
CMD flask run
Build:
Image can be built using a Dockerfile with following command,
docker build -t [username/]<image-name>[:tag] <dockerfile-path>
Example:
docker build -t dockerfile-sample/flask-app:latest .
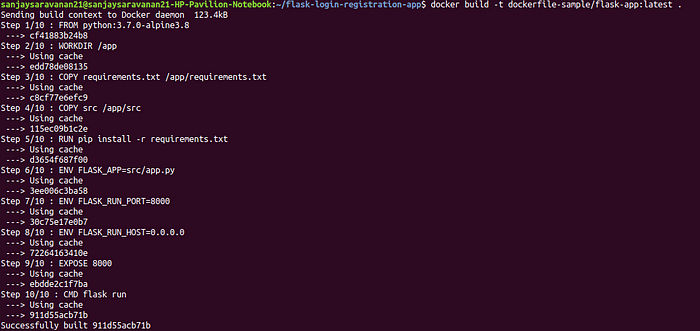
Run:
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
--publish , -p [Host-Port]:[Conatiner-Port]
→Publish a container’s port(s) to the host.
docker run -p 8000:8000 dockerfile-sample/flask-app

Now, the flask application will be running on port 8000 in the local machine.
Reference:
Refer to the following repo https://github.com/sanjaysaravanan/dockerfiles for some sample Dockerfile for Java, Python, NPM applications.
Hope you got the knowledge about containerizing an application. Thank You.